HELLO WORLD!
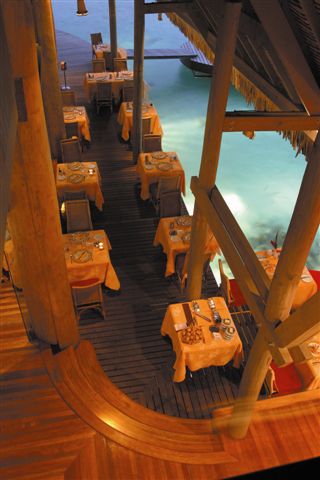
Some random contents
Copyright My Site
Reminder
Your trial membership will expire in 3 days!
revision:
Add <div id="watermark">MESSAGE</div> at the bottom of the page.
Position it accordingly: #watermark { position: fixed; bottom: 0; right: 0; z-index:999; }
To add a watermark to an image, the after pseudo-class can be used:
<img class="watermark" src="IMAGE.JPG"/>
.watermark { position: relative; }
.watermark::after { position: absolute; bottom: 0; right: 0; content: "COPYRIGHT"; }
Some random contents
Your trial membership will expire in 3 days!
<style> .area{width:30vw; height: 40vw; border: 0.2vw solid burlywood;} #watermark{position: relative; bottom: 2vw; right: 1vw; z-index: 999; opacity: 0.5; text-align: right; color: red;} main {user-select: none; -webkit-user-select: none; -moz-user-select: none;-ms-user-select: none;} </style> <div class="area"> <main> <h4>HELLO WORLD!</h4> <img src="../images/7.jpg" width="400" height="400" alt="holidays"/> <p>Some random contents</p> <div id="watermark"> <h4>Copyright My Site</h4> <h4>Reminder</h4> <p>Your trial membership will expire in 3 days!</p> </div> </main> </div>
<div class="area2"> <div class="watermark2">watermark text</div> </div> <style> .area2{width:30vw; height:20vh; border: 0.2vw solid blue;} .watermark2{position:relative; top:1vw; left:2vw; user-select:none; -ms-user-select:none; -moz-user-select:none; -khtml-user-select:none; -webkit-touch-callout:none; -webkit-user-select:none;} </style>
Create a canvas element and image object.
var ctx = document.createElement("canvas").getContext("2d");
var img = new Image();
Add watermark to the image on load.
img.onload = () => {
ctx.drawImage(img, 0, 0, img.naturalWidth, img.naturalHeight);
ctx.fillText("COPYRIGHT", 10, 30);};
Set the image source to start – img.src = "IMAGE.JPG";
<div> <canvas id="demo" class="spec"></canvas> </div> <script> var img = new Image(), watermark = "Copyright", canvas = document.getElementById("demo"), ctx = canvas.getContext("2d"); img.onload = () => { // set image canvas.width = img.naturalWidth; canvas.height = img.naturalHeight; ctx.drawImage(img, 0, 0, img.naturalWidth, img.naturalHeight); // add watermark ctx.font = "bold 2vw Arial"; ctx.fillStyle = "rgba(255, 0, 0, 0.5)"; ctx.fillText(watermark, 20, 70); //download // let anchor = document.createElement("a"); // anchor.href = canvas.toDataURL("image/jpg"); // anchor.download = "demoA.jpg"; // anchor.click(); // anchor.remove(); } // go img.src = "img_flowers.jpg"; </script>
<div> <canvas id="demo-2" class="spec"></canvas> </div> <script> var img1 = new Image(), watermark1 = new Image(), loaded = 0, canvas1 = document.getElementById("demo-2"), ctx_a = canvas1.getContext("2d"); // add watermark when images are fully loaded) function mark () {loaded++; if (loaded==2) { canvas1.width = img1.naturalWidth; canvas1.height = img1.naturalHeight; ctx_a.drawImage(img1, 0, 0, img.naturalWidth, img.naturalHeight); ctx_a.drawImage(watermark1, 0, 0, watermark1.naturalWidth, watermark1.naturalHeight); // donwload (if you want) // let anchor = document.createElement("a"); // anchor.href = canvas.toDataURL("image/jpg"); // anchor.download = "demoB.jpg"; // anchor.click(); // anchor.remove(); }}; // go img1.onload = mark; watermark1.onload = mark; img1.src = "../images/img_flowers.jpg"; watermark1.src = "../images/img_smallflower.jpg"; </script>
This doesn't have any effect on content loaded as part of a browser's user interface, except in textboxes.
values
none : the text of the element and its sub-elements is not selectable. Note that the Selection object can contain these elements.
auto : the used value of auto is determined as follows:
on the ::before and ::after pseudo elements, the used value is none;
if the element is an editable element, the used value is contain;
if the used value of user-select on the parent of this element is all, the used value is all;
if the used value of user-select on the parent of this element is none, the used value is none;
otherwise, the used value is text.
text : the text can be selected by the user.
all : an entire element gets selection; the content of the element shall be selected atomically: if a selection would contain part of the element, then the selection must contain the entire element including all its descendants. If a double-click or context-click occurred in sub-elements, the highest ancestor with this value will be selected.
contain : enables selection to start within the element; however, the selection will be contained by the bounds of that element.
syntax examples
/* Keyword values */ user-select: none; user-select: auto; user-select: text; user-select: contain; user-select: all; /* Global values */ user-select: inherit; user-select: initial; user-select: revert; user-select: revert-layer; user-select: unset;
You should be able to select this text.
Hey, you can't select this text!
Clicking once will select all of this text.
<div class="spec"> <p>You should be able to select this text.</p> <p class="unselectable">Hey, you can't select this text!</p> <p class="all">Clicking once will select all of this text.</p> </div> <style> .unselectable {-webkit-user-select: none; /* Safari */ -ms-user-select: none; /* IE 10+ */ user-select: none;} .all {-webkit-user-select: all; -ms-user-select: all; user-select: all;} </style>
You can't copy me.
But you can accidently copy me (in Safari/WebKit only, not Blink or Gecko) by selecting the periods around me.
.One click selects all this.
<div> <div class="no-copy select"><p>You can't copy me.</p></div> <div class="no-copy select"> . <p>But you can accidently copy me (in Safari/WebKit only, not Blink or Gecko) by selecting the periods around me.</p> . </div> <div class="all-copy select"><p>One click selects all this.</p></div> </div> <style> .select{padding: 2vw; margin: 1vw; background: #eee;} .no-copy p {-webkit-user-select: none; /* Chrome all / Safari all */ -moz-user-select: none; /* Firefox all */ -ms-user-select: none; /* IE 10+ */ user-select: none; /* Likely future */} .all-copy p {-webkit-user-select: all; /* Chrome all / Safari all */ -moz-user-select: all; /* Firefox all */ -ms-user-select: all; /* IE 10+ */ user-select: all; /* Likely future */ } </style>
The userSelect property sets or returns whether the text of an element can be selected or not. If you double-click on some text, it will be selected/highlighted. This property can be used to prevent this.
return the userSelect property : object.style.userSelect;
set the userSelect property : object.style.userSelect = "auto | none | text | all";
auto : default; text can be selected according to the browser's default settings;
none : prevent text selection;
text : the text can be selected by the user;
all : text selection is made with one click instead of a double-click
Click the "try it" button to set the user-select property of the p element to "none":
After you click on the button, it is not possible to select the text of this p element (try to double-click me before and after).
<div class="spec"> <p>Click the "try it" button to set the user-select property of the p element to "none":</p> <button onclick="mySelection()">try it</button> <p id="protect-1">After you click on the button, it is not possible to select the text of this p element (try to double-click me before and after).</p> </div> <script> function mySelection() { var x = document.getElementById("protect-1"); x.style.WebkitUserSelect = "none"; // Safari x.style.msUserSelect = "none"; // IE 10 and IE11 x.style.userSelect = "none"; // Standard syntax } </script>
Click the button to get the value of the "user-select" property of the p element below.
It is not possible to select this text.
<div> <p class="spec">Click the button to get the value of the "user-select" property of the p element below.</p> <button onclick="selectFunction()">try it</button> <p class="spec" id="protect-2" style="user-select:none">It is not possible to select this text.</p> <p class="spec" id="protect-3" style="color:red;"></p> </div> <script> function selectFunction() { document.getElementById('protect-3').innerText = "the above p element is 'user-select:none'" } </script>
The oncontextmenu attribute specifies through the user right-clicks/alt-clicks on an element to open the context menu.It supports all HTML elements.
at the webpage level:
<body oncontextmenu="return false">
JavaScript on page:
document.oncontextmenu = function(){ return false; }
Now try to right click on above red box to open context menu.
<div> <div style="margin-left:3vw;color:white;" oncontextmenu="get()" id="box">Hi there !!</div> <p class="spec">Now try to right click on above red box to open context menu.</p> </div> <style> #box{width: 30vw; height: 10vw; background: blue; border: 0.2vw solid burlywood;} </style> <script> function get() { document.getElementById("box").style.background = "#084C61"; document.getElementById("box").style.color = "darkblue"; alert("you right-clicked the box"); } </script>
The context menu has been disabled on this paragraph.
But it has not been disabled on this one.
<div> <p id="noContextMenu" class="spec">The context menu has been disabled on this paragraph.</p> <p class="spec">But it has not been disabled on this one.</p> </div> <script> const noContext = document.getElementById("noContextMenu"); noContext.addEventListener("contextmenu", (e) => { e.preventDefault(); }); </script>
values:
auto: the element behaves as it would if the pointer-events property were not specified. In SVG content, this value and the value visiblePainted have the same effect.
none: the element is never the target of pointer events; however, pointer events may target its descendant elements if those descendants have pointer-events set to some other value. In these circumstances, pointer events will trigger event listeners on this parent element as appropriate on their way to/from the descendant during the event capture/bubble phases.
SVG only values (experimental):
visiblePainted : SVG only (experimental for HTML). The element can only be the target of a pointer event when the visibility property is set to visible and e.g. when a mouse cursor is over the interior (i.e., 'fill') of the element and the fill property is set to a value other than none, or when a mouse cursor is over the perimeter (i.e., 'stroke') of the element and the stroke property is set to a value other than none.
visibleFill : SVG only. The element can only be the target of a pointer event when the visibility property is set to visible and when e.g. a mouse cursor is over the interior (i.e., fill) of the element. The value of the fill property does not affect event processing.
visibleStroke : SVG only. The element can only be the target of a pointer event when the visibility property is set to visible and e.g. when the mouse cursor is over the perimeter (i.e., stroke) of the element. The value of the stroke property does not affect event processing.
visible : SVG only (experimental for HTML). The element can be the target of a pointer event when the visibility property is set to visible and e.g. the mouse cursor is over either the interior (i.e., fill) or the perimeter (i.e., stroke) of the element. The values of the fill and stroke do not affect event processing.
painted :
SVG only (experimental for HTML). The element can only be the target of a pointer event when e.g. the mouse cursor is over the interior (i.e., 'fill') of the element and the fill property is set to a value other than none, or when the mouse cursor is over the perimeter (i.e., 'stroke') of the element and the stroke property is set to a value other than none. The value of the visibility property does not affect event processing.
fill : SVG only. The element can only be the target of a pointer event when the pointer is over the interior (i.e., fill) of the element. The values of the fill and visibility properties do not affect event processing.
stroke : SVG only. The element can only be the target of a pointer event when the pointer is over the perimeter (i.e., stroke) of the element. The values of the stroke and visibility properties do not affect event processing.
all : SVG only (experimental for HTML). The element can only be the target of a pointer event when the pointer is over the interior (i.e., fill) or the perimeter (i.e., stroke) of the element. The values of the fill, stroke, and visibility properties do not affect event processing.
"pointer-events: none": using this CSS rule on image elements prevents them from responding to click events: right clicking the image will still show a page context menu, but without "save image" or "copy image location" options.
"pointer-events: none" won't stop downloading images using standard browser tools (such as "page info" in Firefox), but should deter visitors with poorer technical skills from downloading images.
A potential downside of this method is that images that don't respond to pointer events can't be used within <a> tags for page navigation: clicking them is ignored. Using the image as the background image of an (inline) block element provides one alternative.
<div class="grid spec"> <div> <ul> <li><a href="https://developer.mozilla.org">MDN</a></li> <li><a href="http://lwitters.com">lwitters.com</a></li> </ul> </div> <style> a[href="http://lwitters.com"]{pointer-events: none;} </style> </div>
Move over the links below to see if the react on pointer events.
<div> <p class="spec">Move over the links below to see if the react on pointer events.</p> <h4>pointer-events: none:</h4> <div class="ex1 spec">Go to <a href="https://www.lwitters.com">my website</a></div> <h4>pointer-events: auto (default):</h4> <div class="ex2 spec">Go to <a href="https://www.lwitters-1.com">my pictures</a></div> </div> <style> div.ex1 {pointer-events: none;} div.ex2 {pointer-events: auto;} </style>